In Java, arrays store multiple values of the same type. You can store numbers, strings, or objects. But before using an array, you must initialize it. That means giving it a size or adding values.
In this article, you will learn how to initialize array Java in five simple ways. Each method is easy to understand and beginner-friendly. We’ll also show short code examples for each method.
1. Initialize Array Using a Fixed Size
This is the most basic way to create an array. You only need to define the size.
Syntax:
javaCopyEditint[] numbers = new int[5];
In this code:
int[]
means the array holds integers.new int[5]
creates space for five numbers.- The default value for each item is 0.
You can assign values like this:
javaCopyEditnumbers[0] = 10;
numbers[1] = 20;
This method is good when you know the size first.
2. Initialize Array with Values (Array Literal)
You can also create and fill an array at the same time.
Syntax:
javaCopyEditString[] fruits = {"Apple", "Banana", "Cherry"};
In this method:
- You don’t need to write the size.
- Java counts the values and sets the size.
You can use this method for small arrays. It’s clean and fast.
Another example:
javaCopyEditint[] ages = {18, 21, 25, 30};
This is the easiest way to initialize array Java with known values.
3. Initialize Array Using a Loop
You can also use a loop to set values. This is useful when the values follow a pattern.
Syntax:
javaCopyEditint[] squares = new int[5];
for (int i = 0; i < squares.length; i++) {
squares[i] = i * i;
}
In this code:
- An array of 5 integers is created.
- The loop fills each slot with the square of the index.
Result:
squares[0] = 0
squares[1] = 1
squares[2] = 4
- and so on.
This method is good when values are generated by a formula or rule.
4. Initialize Array Using Arrays.fill() Method
Java has a built-in method to fill arrays. It’s simple when you want the same value in all spots.
Syntax:
javaCopyEditimport java.util.Arrays;
int[] marks = new int[5];
Arrays.fill(marks, 100);
Here’s what it does:
- Creates an array of size 5.
- Fills all spots with the value
100
.
This is useful for setting default values.
Another example:
javaCopyEditString[] names = new String[3];
Arrays.fill(names, "Unknown");
Now, all names will have "Unknown"
.
This is a fast and clean way to initialize array Java with one value.
5. Initialize Array Using Streams (Java 8 and Above)
Java 8 introduced streams. You can use them to create and fill arrays.
Syntax:
javaCopyEditint[] evenNumbers = java.util.stream.IntStream.range(0, 10)
.filter(n -> n % 2 == 0)
.toArray();
This code creates an array with even numbers from 0 to 9.
Steps in the code:
IntStream.range(0, 10)
makes numbers from 0 to 9..filter(n -> n % 2 == 0)
picks only even numbers..toArray()
turns the stream into an array.
This method is more advanced. But it’s very powerful and compact.
You can also use streams to fill arrays with random numbers:
javaCopyEditint[] randoms = java.util.stream.IntStream.generate(() -> (int)(Math.random() * 100))
.limit(5)
.toArray();
This creates an array of 5 random numbers from 0 to 99.
Summary of Methods
Method | Best For | Code Style |
---|---|---|
Fixed Size | Known size, unknown values | Simple |
Array Literal | Known values at start | Clean and fast |
Loop Initialization | When values follow a pattern | Flexible |
Arrays.fill() | Filling with one value | Short and clean |
Java Streams | Complex generation and filters | Modern and powerful |
Which Method Should You Use?
It depends on your use case.
- Use fixed size when size is known but values are set later.
- Use literals when you know values ahead of time.
- Use a loop for patterns or formulas.
- Use Arrays.fill() for same-value arrays.
- Use streams when you want smart or dynamic arrays.
Each method has its own use. Choose the one that fits your task best.
Best Practices
Here are some tips when you initialize array Java:
- Always choose the right array type (
int[]
,String[]
, etc.). - Avoid magic numbers. Use variables for size.
- Don’t forget index starts at 0.
- Be careful not to go out of bounds.
- Use enhanced for-loops to read arrays:
javaCopyEditfor (int num : numbers) {
System.out.println(num);
}
This loop is simple and avoids errors.
Final Words
Arrays are an important part of Java. You’ll use them in many programs. Knowing how to initialize array Java is a key skill.
This article showed 5 simple ways:
- Fixed size
- With values
- Using loops
- With
Arrays.fill()
- With Java streams
Each method is useful in different cases. Practice them to understand better. Once you get the idea, using arrays becomes very easy.
Arrays are the foundation for many data structures. So it’s important to master them early. Happy coding!
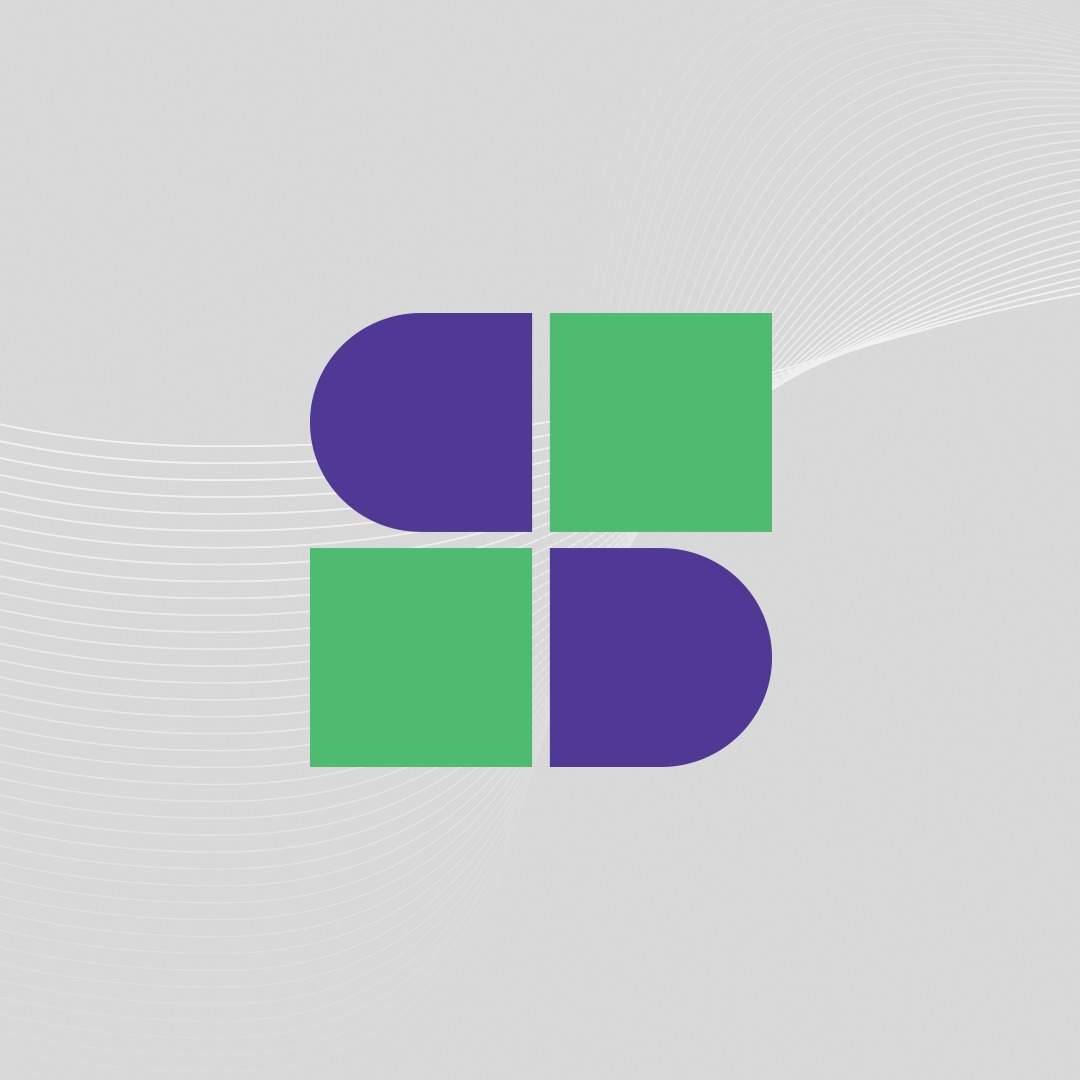
Software Testing Lead providing quality content related to software testing, security testing, agile testing, quality assurance, and beta testing. You can publish your good content on STL.